(Perl:
Lesson 5)
{ Writing Basic
Subroutines }
Section 0. Background
Information |
- What is Perl
- Perl is a high-level, general-purpose,
interpreted, dynamic programming language. Perl was originally developed
by Larry Wall in 1987 as a general-purpose Unix scripting language to
make report processing easier. Since then, it has undergone many
changes and revisions and become widely popular amongst programmers.
Larry Wall continues to oversee development of the core language, and
its upcoming version, Perl 6. Perl borrows features from other
programming languages including C, shell scripting (sh), AWK, and sed.
The language provides powerful text processing facilities without the
arbitrary data length limits of many contemporary Unix tools,
facilitating easy manipulation of text files. Perl gained widespread
popularity in the late 1990s as a CGI scripting language, in part due to
its parsing abilities.
- Getting Perl
- For the purposes of these perl lesson, I
will be using a perl package that comes standard on Backtrack, Ubuntu
and most flavors of Linux and Unix.
- However, if you are using Windows, instead
of a Linux, Unix or MAC operating system, you still have options.
- Pre-Requisite
-
Lab
Notes
- In this lab we will do the following:
- We will download a basic program that
contains subroutines.
- We will analyze the program line by
line.
- We will create a program that contains
the following elements:
- subroutine, standard input, and an
IF/ELSE clause.
- Legal Disclaimer
- As a condition of your use of this Web
site, you warrant to computersecuritystudent.com that you will not use
this Web site for any purpose that is unlawful or
that is prohibited by these terms, conditions, and notices.
- In accordance with UCC § 2-316, this
product is provided with "no warranties, either express or implied." The
information contained is provided "as-is", with "no guarantee of
merchantability."
- In addition, this is a teaching website
that does not condone malicious behavior of
any kind.
- Your are on notice, that continuing
and/or using this lab outside your "own" test environment
is considered malicious and is against the law.
- © 2013 No content replication of any
kind is allowed without express written permission.
Section 1.
Login to BackTrack |
- Start Up VMWare Player
- Instructions:
- Click the Start Button
- Type Vmplayer in the search box
- Click on Vmplayer
-
- Open a Virtual Machine
- Instructions:
- Click on Open a Virtual Machine
-
- Open the BackTrack5R1 VM
- Instructions:
- Navigate to where the BackTrack5R1 VM
is located
- Click on on the BackTrack5R1 VM
- Click on the Open Button
-
- Edit the BackTrack5R1 VM
- Instructions:
- Select BackTrack5R1 VM
- Click Edit virtual machine settings
-
- Edit Virtual Machine Settings
- Instructions:
- Click on Network Adapter
- Click on the Bridged Radio button
- Click on the OK Button
- Play the BackTrack5R1 VM
- Instructions:
- Click on the BackTrack5R1 VM
- Click on Play virtual machine
-
- Login to BackTrack
- Instructions:
- Login: root
- Password: toor or <whatever you changed
it to>.
-
- Bring up the GNOME
- Instructions:
- Type startx
-
Section 2.
Bring up a
console terminal |
- Start up a terminal window
- Instructions:
- Click on the Terminal Window
- Obtain the IP Address
- Instructions:
- ifconfig -a
- Note(FYI):
- My IP address 192.168.1.111.
- In your case, it will probably be
different.
-
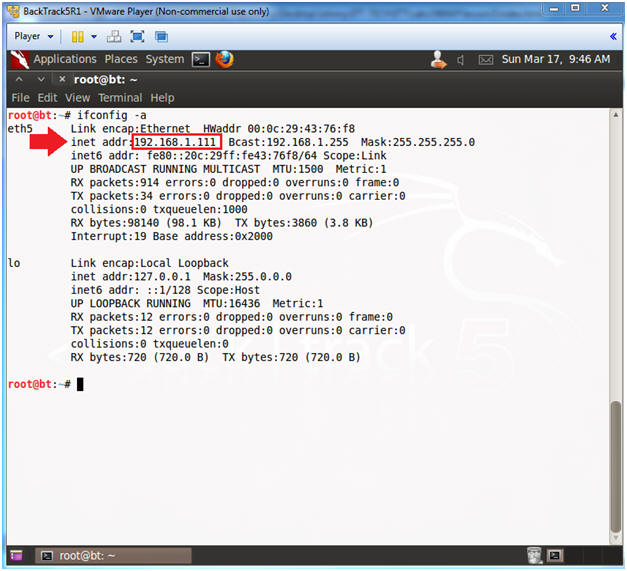
Section 3.
Download lesson5a.pl |
- Become the student user and make a directory
- Instructions:
- su - student
- mkdir -p perl_lessons
- cd perl_lessons
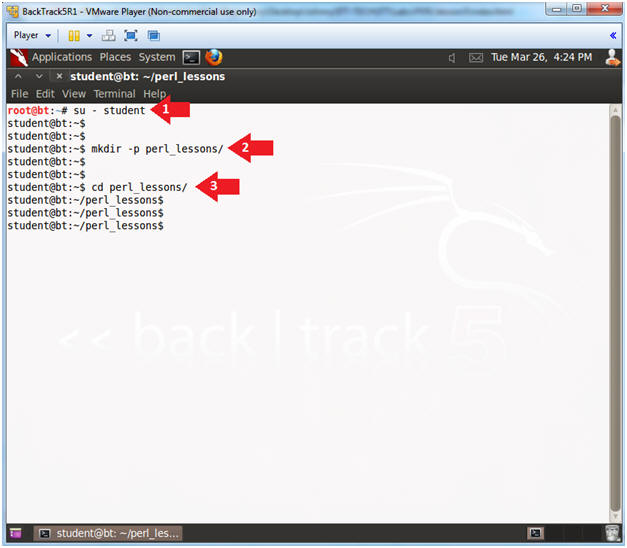
- Download lesson5a.pl
- Instructions:
- wget http://www.computersecuritystudent.com/UNIX/PERL/lesson5/lesson5a.pl
- chmod 700 lesson5a.pl
- perl -c lesson5a.pl
- Run lesson5a.pl
- Instructions:
- ./lesson5a.pl
- Note(FYI):
- Before continuing to the proof of lab
section.
- Read each line of the code and examine
how each subroutine executes.
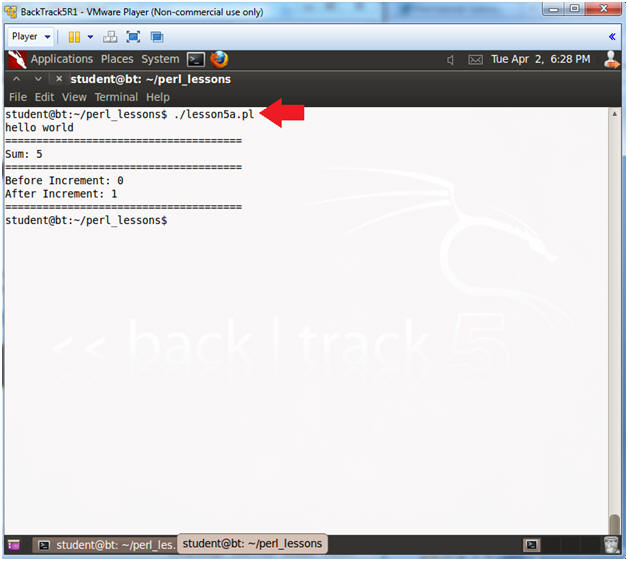
Section 6.
Analyze The Code |
- SheBang Directive
- Instructions:
- head -1 lesson5a.pl
- Note(FYI):
- #! - is called the SheBang Directive.
SheBang is an interpreter directive that tells Linux to load the
following program.
- /usr/bin/perl - is the Perl
Interpreter. SheBang tells the program loader to run the Perl
Interpreter.
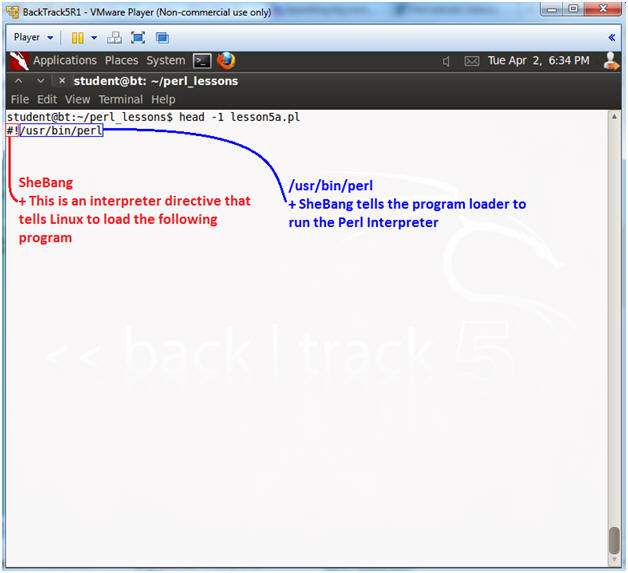
- Explaining Lines 3 through 19
- Instructions:
- vi lesson5a.pl
- :set nu
- Note(FYI):
- Lines 4, 7, 10, 13, 16, and 19 are all
subroutines.
- A subroutine is a user define function
to execute a set of programmable actions.
- Subroutine &print_it() accepts a string
surrounding by quotes.
- Subroutine &add_it() accepts two
numbers and adds them to create a sum.
- Subroutine &increment_it() accept one
number and increments it by one digit.
- Explaining Lines 22 through 29
- Instructions:
- Arrow down to line 22
- Note(FYI):
- Line 22: sub print_it
- print_it is the name of the
subroutine.
- Line 23: {
- Start of the print_it subroutine
- Line 29: }
- End of the print_it subroutine.
- Line 25: chomp(my $tmp = $_[0]);
- Variable $tmp is assigned the input
of the first parameter. Notice $_[0] is the first
parameter.
- Line 28: print "$tmp\n";
- Print the contents of the variable
$tmp.
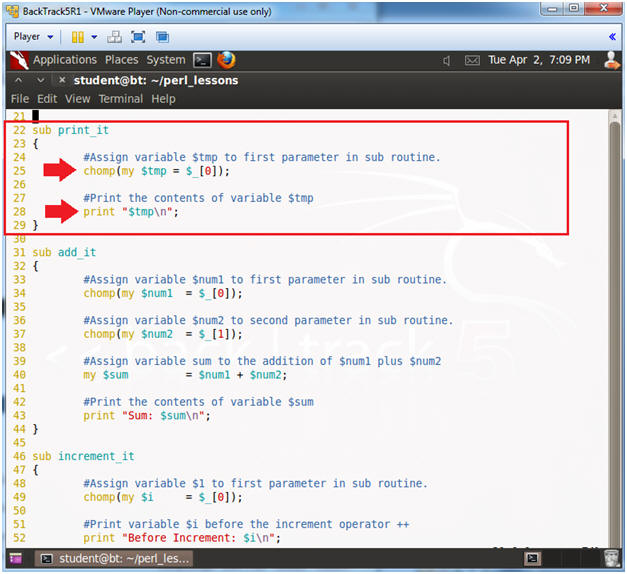
- Explaining Lines 31 through 43
- Instructions:
- Arrow down to line 31
- Note(FYI):
- Line 31: sub add_it
- add_it is the name of the
subroutine.
- Line 32: {
- Start of the add_it subroutine
- Line 44: }
- End of the add_it subroutine.
- Line 34: chomp(my $num1 = $_[0]);
- Variable $num1 is assigned the
input of the first parameter. Notice $_[0] is the first
parameter.
- Line 37: chomp(my $num2 = $_[1]);
- Variable $num2 is assigned the
input of the second parameter. Notice $_[1] is the second
parameter.
- Line 40: my $sum = $num1 + $num2;
- The variable $sum is the summation
of $num1 plus $num2.
- Line 43: print "Sum: $sum\n";
- Print the contents of the variable
$sum.
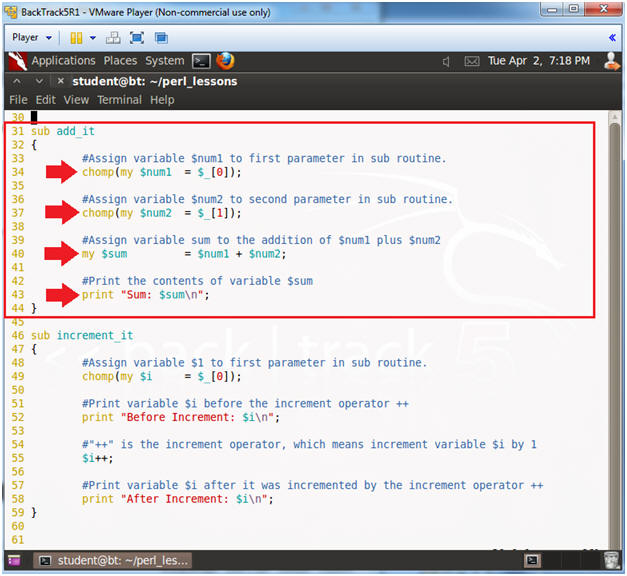
- Explaining Lines 46 through 59
- Instructions:
- Arrow down to line 46
- Note(FYI):
- Line 46: sub increment_it
- increment_it is the name of the
subroutine.
- Line 47: {
- Start of the increment_it
subroutine
- Line 59: }
- End of the increment_it subroutine.
- Line 49: chomp(my $i = $_[0]);
- Variable $i is assigned the input
of the first parameter. Notice $_[0] is the first
parameter.
- Line 52: print "Before Increment: $i\n";
- Print the contents of the variable
$i before the increment
variable is executed.
- Line 55: $i++;
- For our purposes, the increment
operator
(++),
increment variable $i by 1 digit.
- Line 58: print "After Increment: $i\n";
- Print the contents of the variable
$i after the increment
variable is executed.
- Save and Quit
- Instructions:
- Press the <Esc> key
- :q!
- Press the <Enter> key
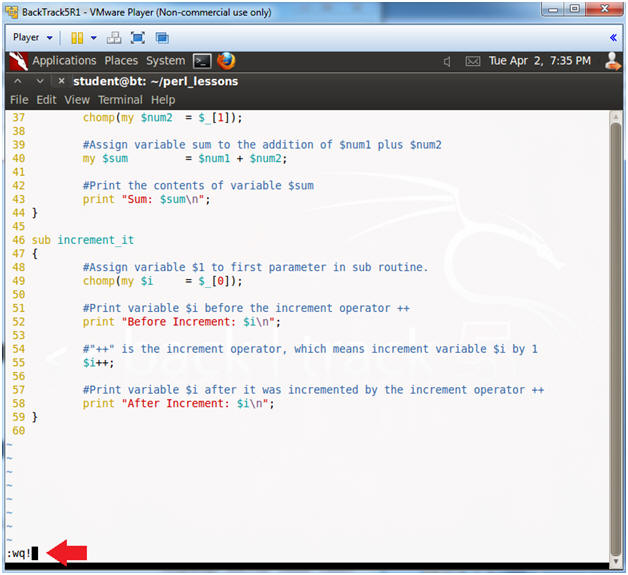
- Project
- Instructions:
- Write a program called lesson5b.pl
- The program should contain a subroutine
called search_string
- Remember your subroutine should start
with a {
and end wish a }.
- The program should prompt the user to
supply a string.
- e.g., print "Supply String: ";
- The program should assign variable
$tmp to standard input
- e.g., chomp(my $tmp = <stdin>);
- The program should contain an IF/ELSE
statement that performs the following test
-
IF variable $tmp contains
the string "student",
THEN print Match Found,
ELSE,
Match Not Found.
- e.g., if($tmp =~ m/student/i)
- After you build your subroutine, you
need to call it, by placing the following statement before the
actual subroutine structure.
- Proof of Lab
- Instructions
- chmod 700 lesson5b.pl
- perl -c lesson5b.pl
- ./lesson5b.pl
- ./lesson5b.pl
- date
- echo "Your Name"
- Put in your actual name in place of
"Your Name"
- e.g., echo "John Gray"
- Do a PrtScn, Paste into a word
document, and upload to Moodle.
-
Proof Of Lab
Instructions:
- Press the PrtScn key
- Paste into a word document
- Upload to Moodle
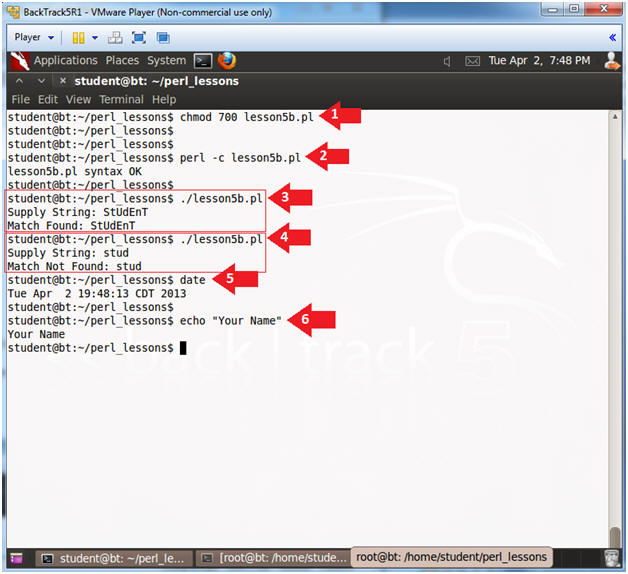
|

 
|